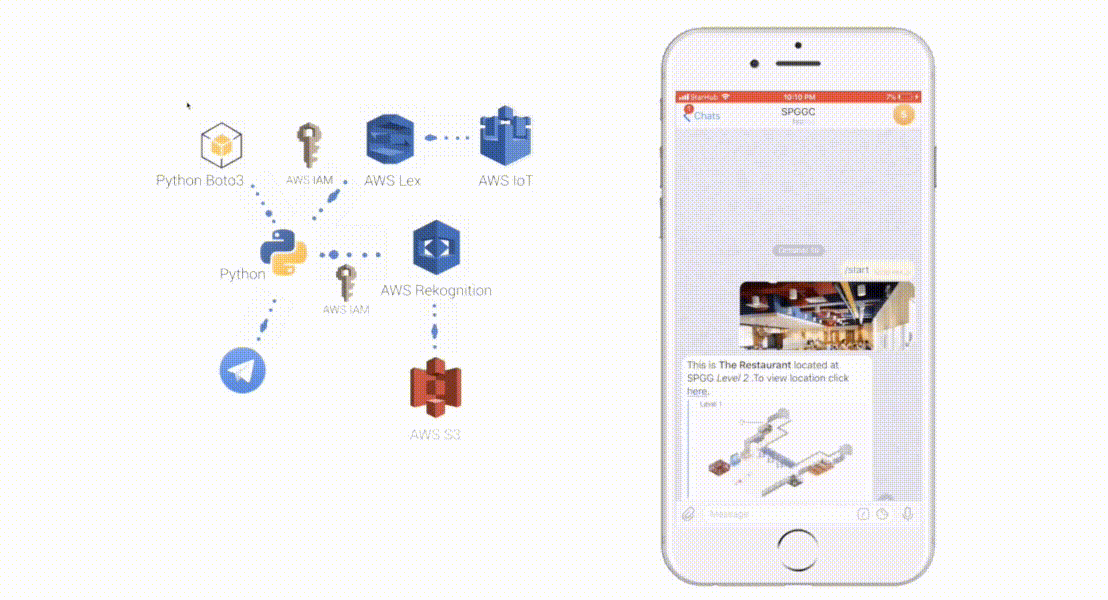
SPGGC
SPGGC is a Telegram chatbot powered by AWS technologies, designed to help users check facility crowd levels, monitor real-time temperatures, and identify locations within the Singapore Polytechnic Graduates Guild (SPGG). Utilizing AWS IoT, the chatbot integrates with IoT sensors to provide users with accurate, up-to-date information, making it easier to plan their visits to various SPGG facilities.
Problem
Human error and carelessness is inevitable, yet it may cost a fortune of repair, mitigate, or prevent. Many organizations and establishments around Singapore using cloud services has also driven competition for whomever can operate more efficiently as well as who can offer better and faster services to customers. To keep up with the demand in services, the solution is an open platform cloud service with multiple IoT devices scattered around SPGG capable of filling-in human shortcomings as well as offer satisfying and swift services.
Idea
The SPGGC project aims to enhance the user experience at Singapore Polytechnic Graduates Guild (SPGG) by leveraging IoT and AWS technologies through a Telegram chatbot. The chatbot allows users to check real-time crowd levels at various facilities, monitor temperature conditions, and identify specific locations within SPGG. This provides users with convenient, actionable information before visiting, making the guild smarter and more efficient.
Solution
Amazon Web Services:
-
Lambda
-
S3
-
DynamoDB
-
SNS
-
IoT
-
Lex
-
Rekognition
-
IAM
Open-source Protocol:
-
MQTT
Tech:
-
NodeMCU
-
Temp Sensor
-
Echo Dot
Phase 1 - Integration of IoT device at SPGG with AWS Services

NodeMCU as a way to obtain WiFi connectivity enabling them to connect to the cloud. It is more likely that the WiFi would fail than AWS.
IP rating is also known as International Protection ratings. These standards are used to define the levels of sealing effectiveness of electrical enclosures against intrusion from foreign bodies such as dirt and water. For all our sensors we use IP65 boxes to shield it from dust and allow for high resistance to water exposure enabling our sensors to function in Singapore’s climate and environment. The batteries we use are 3.7v lithium ion batteries used to power our sensors and are small enough to be implanted within the IP65 boxes. The underground water leakage detector uses a soil moisture called ESPM30421S. The sensor has a build in potentiometer which would enable us to set the threshold for determining how wet should it be when HIGH or LOW is triggered.
The temperature sensor uses DS18B20 1-Wire Digital Thermometer to collect data from the surroundings. Stainless steel tube 6mm diameter by 30mm long. It has a usable temperature range of -55 °C to 125 °C and query time of less than 750 ms.
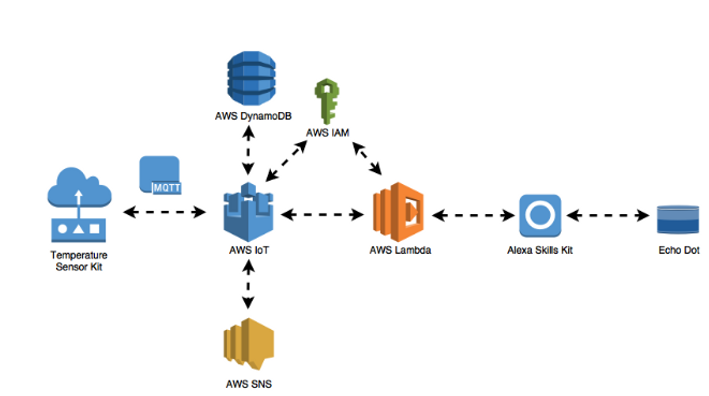
The temperature sensor will generate values, which will be sent to AWS IoT through the Amazon IoT client. In AWS IoT, the temperature values will be displayed and updated under a shadow. The shadow provides a persistent representation of our temperature sensor in the cloud, allowing it to continually publish the current temperature values. These values can then be used by applications or other devices. If the shadows are connected successfully, they will generate metadata. In this case, the generated metadata is:
json
{
"metadata": { "reported": {
"temperature": {
"timestamp": 1517673840
},
"fahrenheit": {
" timestamp": 1517673840
},
"id": {
"timestamp": 1517673840
}
},
"timestamp": 1518021488, "version": 1184
}
}
Once in the AWS IoT shadow, AWS IoT can push the data to a NoSQL database service called AWS DynamoDB. AWS DynamoDB is a fully managed cloud database, which we use to store all generated temperature values. The table is created with a primary partition key of stamps (String) and a primary sort key of temperature (String). We encountered an issue where AWS DynamoDB was not updating. This was due to an incorrect AWS IoT shadow query. Both the Hash Key and Range Key must match and be in the correct format.
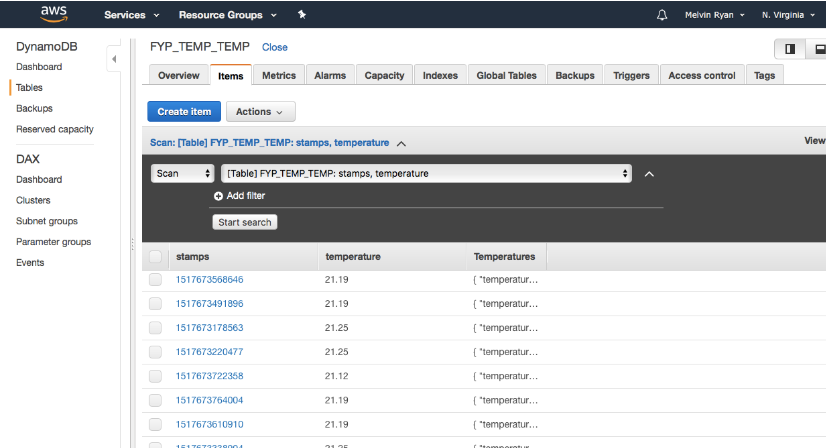
AWS IoT is able to send an alert notification through Amazon Simple Notification Service (AWS SNS). AWS SNS is a mobile notifications that allows us to fan-out messages to a large pool of subscribers and mobile devices. In this case, we are using SNS to send alert notification which allows SPGG admin to receive an alert if a particular venue exceed or below threshold
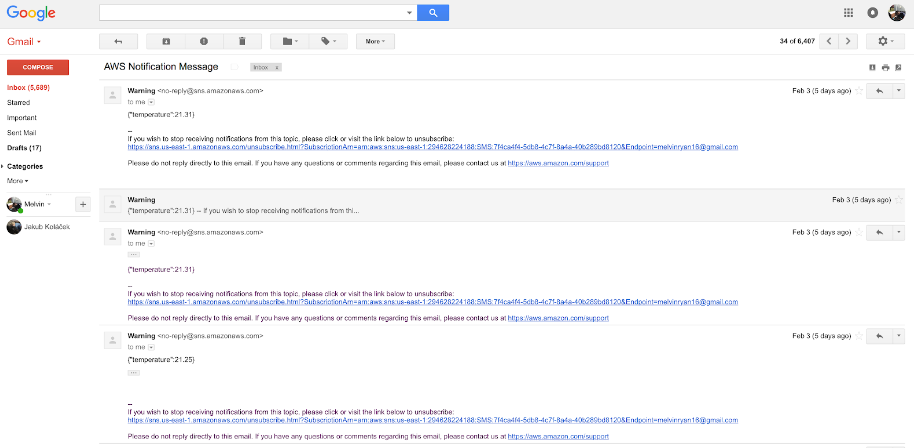
AWS IoT is able to send an alert notification through Amazon Simple Notification Service (AWS SNS). AWS SNS is a mobile notifications that allows us to fan-out messages to a large pool of subscribers and mobile devices. In this case, we are using SNS to send alert notification which allows SPGG admin to receive an alert if a particular venue exceed or below threshold
AWS IAM is integrated between AWS IoT and AWS Lambda. AWS IAM, which stands for ‘Amazon Identity and Access Management,’ enables me to securely control access to resources and services. In this case, I have granted AWS Lambda permission to access AWS IoT.
In AWS Lambda, there is a Node.js code that allows data generated by the temperature sensor kit to be readable by Alexa, which will be used by a voice-controlled device like the Echo Dot. Users are able to ask for the current temperature at a specific venue.
Below is an example of part of the code deployed using AWS Lambda. The AWS SDK is required in the program. The AWS SDK is a library that allows the program to recognize AWS commands. For example, AWS.config.region is used to declare the AWS IoT region, which in this case is us-east-1, and the endpoint is the AWS IoT endpoint generated by the AWS IoT topic.
var AWS = require('aws-sdk'); AWS.config.region = config.IOT_BROKER_REGION; // Initializing client for IoT
var iotData = new AWS.IotData({endpoint: config.IOT_BROKER_ENDPOINT});
There are three types of requests required in this case: LaunchRequest, IntentRequest, and SessionEndedRequest. LaunchRequest is where the Echo Dot greets the user: "Welcome to Singapore Polytechnic Graduates Guild, what can I help you with?" IntentRequest is a request made by the Alexa Skills Kit.
if (event.request.type === "LaunchRequest") {
onLaunch(event.request,
event.session,
function callback(sessionAttributes, speechletResponse) {
context.succeed(buildResponse(sessionAttributes, speechletResponse));
});
} else if (event.request.type === "IntentRequest") {
onIntent(event.request, event.session,
function callback(sessionAttributes, speechletResponse) {
context.succeed(buildResponse(sessionAttributes, speechletResponse)); });
} else if (event.request.type === "SessionEndedRequest") {
onSessionEnded(event.request, event.session);
context.succeed();
}
Phase 2 - Crowd Information
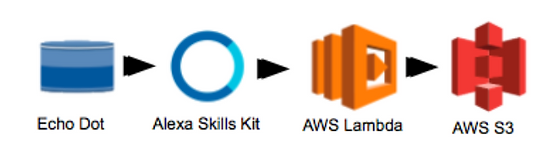
The aim of this project is to make the Singapore Polytechnic Graduates Guild (SPGG) a smarter guild. There are more than five facilities available at SPGG. For example, consider the gymnasium. Wouldn’t it be better for SPGG members to ask how many people are currently at the gym before deciding to go? In this scenario, I am using three main AWS services: Alexa Skills Kit, AWS Lambda, and AWS S3.
AWS Lambda contains the AWS SDK, which allows me to use AWS commands and intents. AWS Lambda will help the Alexa Skills Kit access the AWS S3 bucket containing a CSV file. This CSV file holds a list of the number of people at each venue. The following part of the code instructs AWS Lambda to access AWS S3 and retrieve the contents of the bucket, specifically the dataset.csv file.
var s3 = new AWS.S3();
var params = { Bucket: 'schinfo', Key: 'dataset.csv' }
If the first element of the row array, after removing apostrophes ("), is equal to the intent MyStatess:
for (var i = 1; i < dataArray.length; i++) {
var rowArray = dataArray[i].split(',');
if (rowArray[0].replace(/"/g,"") == myStatess) {
venue = rowArray[2]; say = "The operating hours of " + myStatess + " is from " + venue;
if (!sessionAttributes.requestList) {
sessionAttributes.requestList = [];
}
}
}
The Alexa Skills Kit contains intents, slot types, and sample utterances. Intents are used to trigger the keyword and topic of the conversation. Slot types contain a list of venues such as the gymnasium, bowling alley, tennis courts, etc. Finally, sample utterances are the preset questions that the Echo Dot will recognize. For the integration between the Alexa Skills Kit and AWS Lambda to work, the intents and AWS Lambda ARN must match.
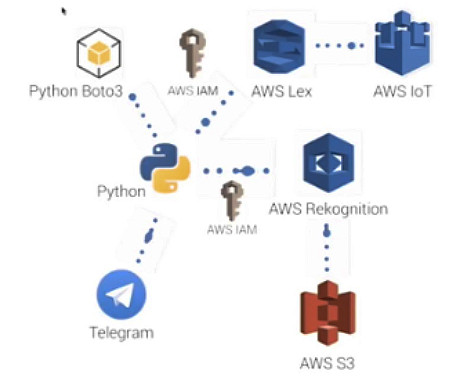
AWS Rekognition is an artificial intelligence service by Amazon Web Services. In this implementation, I am using a Telegram chatbot. Telegram offers an open-source chatbot, and while there are various open-source platforms such as Facebook, Spark, and Discord, I chose Telegram due to the availability of its library and extensive documentation from other sources. I programmed the chatbot in Python, using an IDE that has boto3, the AWS SDK for Python.
Final Outcome
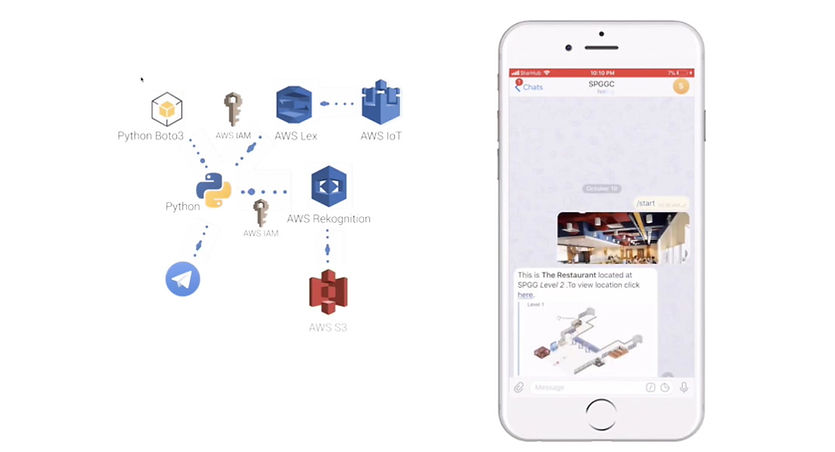